Events in Web Forms work somewhat differently than events in traditional client forms or in client-based Web applications. The difference lies primarily in where the event is handled.
In client-based applications, events are raised and handled on the client. In Web Forms pages, on the other hand, events associated with server controls are raised on the client but are handled on the Web server. An ASP.NET page raises events on the server, though, as do certain controls, such as the Calendar and AdRotator Web controls.
NOTE: Page events are covered in Web Forms Page Processing Stages. The remainder of this document focuses on events and event handling in Web Forms controls.
For events raised on the client, the Web Forms event model requires that the event information be captured on the client and an event message transmitted to the server. Because of how the Web works, this can only happen via an HTTP post. The page framework must interpret the post to determine what event occurred, and then call the appropriate method in your code to handle the event.
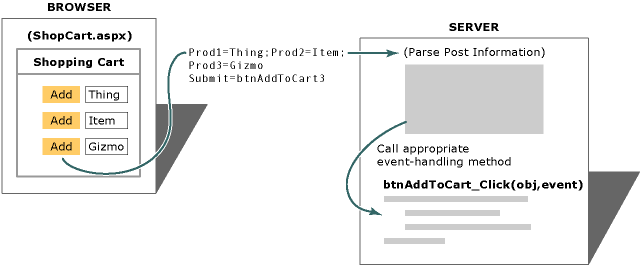
ASP.NET Server Controls Event Model
ASP.NET handles virtually all of the mechanics of capturing, transmitting, and interpreting the event. This allows you to focus more on how to handle the event, instead of how the event information is made available to your code. Even so, there are certain aspects of event handling in Web Forms pages that you should be aware of.
Because most Web Forms events require a round trip to the server for processing, they can affect the performance of a form. For this reason, server controls offer a limited set of built-in events, which are mostly click-type events.
The following examples demonstrate how you can specify and code a handler for the click events of various button controls.
The last two examples illustrate handling click events of child controls defined within a templated control, whch we shall be covering in a while.
Some server controls support a special version of a change-type event, which is raised when a control's value changes. For example, the CheckBox and RadioButton Web server controls raise a change event whenever the user clicks the box, while the DropDownList and ListBox controls raise a change event whenever the user selects a different list item.
The following examples demonstrate how you can specify and code a handler for the change events of various server controls.
The intrinsic client-side events defined in HTML 4.0 are not supported for server controls. These events, though, can still be handled using client-side methods.
NOTE: ADO.NET supports a separate set of events that are specific to data handling. These events are covered in a separate section.
Web Forms server controls follow a standard pattern for handling information relevant to each event, which is made available to the designated event handler. By convention, all events pass two arguments:
- an object referencing the source that raised the event, and
- an event object containing data for the event.
The second argument is usually of type System.EventArgs, but for control events that generate data, is of a type specific to that control.
For example, a Button Web server control supports both a Click event as well as a Command event. Because Click events do not generate data, the second argument for the event is of type EventArgs, whereas for the Command event is of type CommandEventArgs, which includes information about the command name and other event data.
This event object can be accessed dynamically, and provides a convenient way to determine specific event attributes, such as which control caused the event, in which row or item the event occurred, etc., and lets us use these values for whatever purposes we can imagine.
For information on specific event arguments, see the reference documentation for the control event you are working with.
In Web server controls, only certain events, typically click events, cause the form to be posted back to the server. Change events in both HTML and Web server controls are captured but do not immediately cause a post. Instead, they are cached by the control until the next time a post occurs. Then, as the page is processed on the server again, all pending events are raised and processed.
During server page processing, all change events are processed first, in no particular order. After which, the click event that caused the form to be posted is processed.
NOTE: You should not create application logic that relies on the change events being raised in a specific order unless you have detailed knowledge of page event processing.
You can specify that a change event should cause a form post. Web server controls that support a change event include an AutoPostBack property, which when set to true, causes the form to post immediately when the control's change event occurs.
For example, by default, a DropDownList control's SelectedIndexChanged event does not cause the page to be submitted. By setting the control's AutoPostBack property to true, though, you specify that as soon as a user changes a selection, the page is sent to the server for processing.
The following examples demonstrate using AutoPostBack in conjunction with the change events of various server controls.
IMPORTANT: AutoPostBack requires that the user's browser be set to allow scripting. While this is the default in most cases, some users may have scripting disabled for security reasons. For more information, see Web Forms Controls and Browser Capabilities.